Before I start telling you how to build your docker container with NodeJS application, let me tell you why you would need a container for the blockchain.
As you know, blockchain is a decentralized ecosystem where you can expect several nodes are running in different part of the region or places. But as a developer when you are building your decentralized network you might find it difficult to set up and purchase so many hosts or nodes to test your decentralized application.
Since container has the strip down version of the OS and essential utilities along with the developed application, it could replicate the same idea of the decentralization without any dedicated servers or virtual machines. You could run more than 100 docker containers within your same computer.
In this article, I will try to explain how you can create a straightforward NodeJS server-based application and containerized it through the docker. This article does not contain blockchain application at this moment but in future of my writings, I would definitely explain how you would containerize the blockchain application. Please check my previous article in which I have mentioned how you can run a private blockchain node in a container http://blockchainsfalcon.com/running-a-private-blockchain-node/.
Building a NodeJS Application:
The application that I am intended to develop is nothing more than a small server which will listen to the port 5000 for the HTTP request. For the code development, I am using PHPStorm IDE , but you can use visual code or any other editor which you like.
- Create a new empty project.
- Create a new JS file as “Server.js”
- Put the server code inside the file “Server.js” , this will contain our application listening code and, it will display three different pages on the web.
/*** Created by farhanx on 7/29/2018. */ 'use strict'; const express = require('express'); // Constants const PORT = 5000; // App const app = express(); app.get('/', function (req, res) { res.send('Welcome to BlockchainsFalcon\n'); }); app.get('/dashboard', function (req, res) { res.send('Dashboard page\n'); }); app.get('/blockchain', function (req, res) { res.send('blockchain page\n'); }); app.listen(PORT); console.log('Running on http://'+HOST+':'+PORT);
4 – Create a Package.json file and add the dependencies as below code. This code will contain the default start command which will automatically select the “server.js” file as the main caller.
{
"name": "farhan_dapp_starter",
"version": "1.0.0",
"description": "Node.js on Docker",
"author": "Farhan Khan",
"main": "server.js",
"scripts": {
"start": "node server.js"
},
"dependencies": {
"express": "^4.16.1"
}
}
5 – Now call the “npm install” this will add all the required dependencies. Npm is the NodeJS package manager just like PHP’s composers.
6 – After npm frees from its activity, then a new folder and file will be appeared “node_modules” and “package-lock.json.” The new folder contained all the essential dependent libraries which are required for any NodeJS application to run.
7 – Now run and test it using this command “nodejs server.js”. To see the result, you must use http://localhost:5000 and use sub-paths like “/dashboard” or “/blockchain” will show other pages. Congratulation your NodeJS app is now ready.
Containerize the App:
It is must to create the docker file inside the same directory. “Dockerfile” remember there is no extension require in this file. Now write the docker as below:
FROM node:8 # Create app directory WORKDIR /usr/src/app # Install app dependencies # A wildcard is used to ensure both package.json AND package-lock.json are copied # where available (npm@5+) COPY package*.json ./ RUN npm install # If you are building your code for production # RUN npm install --only=production # Bundle app source COPY . . EXPOSE 5001 CMD [ "npm", "start" ]
The Docker file starts with “FROM” keyword which denotes that the new image must be downloaded from the docker hub with a latest Node JS docker’s image. The second command in the Dockerfile instruct to create an app folder and afterward copy all the package files into the folder.
The npm command instructs to install all the dependent libraries of NodeJS. The copy command copy all the app sources. EXPOSE mean the port on which the NodeJS App would be available through a browser. The last instruction force the process to run the default file which in our case is server.js.
Open Docker QuickStart Shell
If you are using docker toolbox on the windows, then open the Docker Quickstart shell and move to the app directory where you have previously created the Dockerfile.
Directly build the docker file with build command as below.
$ docker image build -t farhan/mynode .
Once it is built, you can run the container using the run command by mapping the internal nodeJS server’s port to the external exposed port which you have mentioned inside the Dockerfile.
$ docker run -p 5001:5000 farhan/mynode
Running above command will take you inside the server.js process, and you will be started seeing
docker_web_app@1.0.0 start /usr/src/app
> node server.js
Running on http://localhost:5000
You can exit from this interactive console without closing the container by pressing “CTRL+C” on the windows shell or quick start docker terminal. One thing you should always remember, the port you are using inside the App should be mapped to the container’s port which will expose the App. That is why the App will be appeared in the browser by using the exposed port instead of the internal App’s port which is now running inside the container.
Another exciting thing about the “run” command is it can also randomly generate names for your container as well, in my case dazzling_ardinghelli is the name created by the docker for my container.
To see if your docker is running fine check the existing running containers by ps command as below.
$docker ps
It will list the docker as below

Congrats your application is not containerized and you can access in windows using default machine with IP : http:// 192.168.99.100:5001
As previously mentioned, It is very crucial to test your blockchain network with multiple nodes. Therefore, the best way is to create multiple Ethereum or hyper ledger’s nodes in the containers. Above steps are sample steps to convert any application into a container.
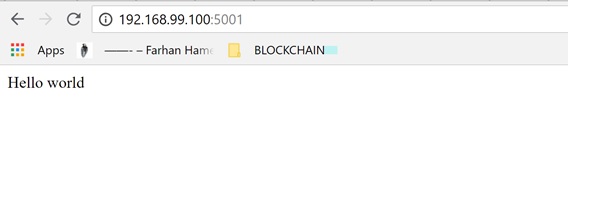